Hello
My question is how do i load a NBT structure with ConfigurationNode and navigate its tree.
So i have been trying to load an NBT file using ConfigurationNode.
but i have no idea how to navigate the tree. this is where i want to get to in the tree.
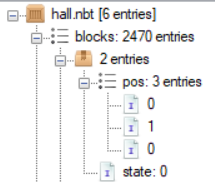
Is there a key for this and how am I going to index over the blocks with that key
I know it loads and converts it because I can use getString() and it renters a string with all the data.
I can see the block pos and state data in the string.
public static ConfigurationNode readNBT(Path path) {
System.out.print(path);
try (InputStream inputStream = Files.newInputStream(path)) {
DataContainer dataContainer = DataFormats.NBT.readFrom(inputStream);
final DataTranslator<ConfigurationNode> translator = DataTranslators.CONFIGURATION_NODE;
final DataView container = dataContainer;
return translator.translate(container);
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
Is there a reason why you need it as a ConfigurationNode
? The DataView
interface is probably best here.
If you do need to use a configuration node, then getNode()
, getChildrenList()
and getChildrenMap()
are what you’re looking for.
Otherwise in a DataView
, you use a DataQuery
to specify a path in the structure.
This is my problem the with DataView
//In this case build is a DataView
objected
build.getList(DataQuery.of(“blocks”)) ;
I now have a list of entries that contain an int array and int the array is the pos and the int is the state.
But because the list is type Object this gives me some problems for 2 reasons.
- I don’t know what to cast this to to make it usable
2.How do I get the 2 items contained in side of each entry
Just the way this works i don’t think a key will work because each block has its own propertys
and in this case there are 2470 blocks and they are all in the same place with no IDs. as I understand it a key only works on a node with no other node that are the same a it. example there are 2470 blocks each has a pos now dose the key know which one I want.
Blockquote ConfigurationNode build = NBTReader.readNBT(Paths.get(“hall.gunzip”));
//This will return 0
build.getChildrenList().size();
//but this will return a string of the whole file tree
build.getString();
What do I even pass in here getNode()
;
I could just maybe use the string data but there must be a better way.
Note the nbt files are compressed and need to be unziped before they even load.